How to Build an AI Investing Bot: A Step-by-Step Guide to Automated Trading
2024-10-06
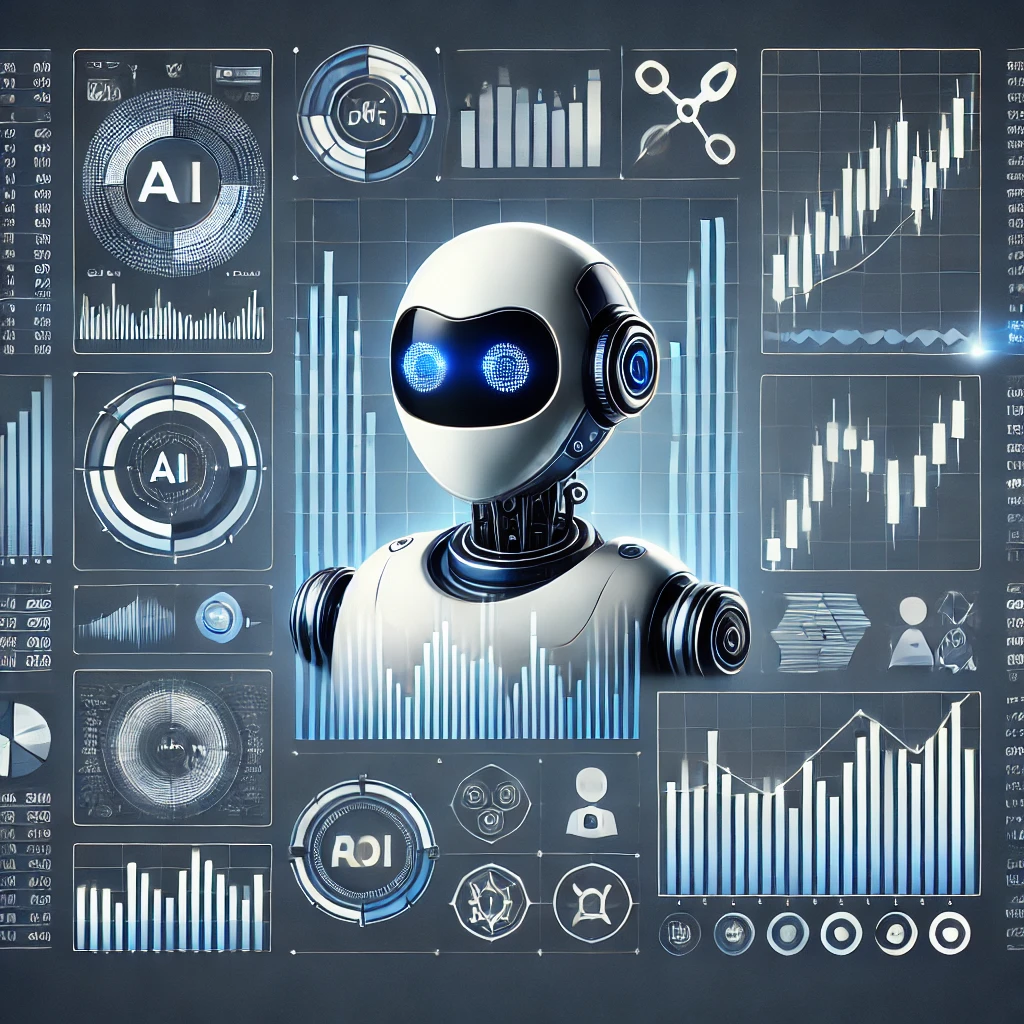
Introduction
In today’s fast-paced financial markets, automating your trading strategy can give you a competitive edge. AI-driven trading bots can analyze vast amounts of data, execute trades faster than any human, and take advantage of short-term price fluctuations or long-term market trends. This guide will walk you through building an AI-powered investing bot that automates both day trading and long-term investment strategies. We'll cover key trading algorithms, connecting to the Interactive Brokers API, and how to integrate GPT-4 for AI automation.
Whether you're a day trader or a long-term investor, this tutorial will help you streamline your trading strategy and leverage the power of AI.
1. Common Trading Strategies: Day Trading vs. Long-Term Trading
Before we dive into building the bot, it's essential to understand the difference between day trading strategies and long-term investment strategies:
Day Trading Strategies
Day traders focus on capitalizing on short-term price movements, often executing multiple trades in a single day. Common day trading strategies include:
- Scalping: Profiting from small price gaps or inefficiencies in the market.
- Momentum Trading: Buying or selling based on the strength of market movements.
- Range Trading: Buying at support levels and selling at resistance levels within a defined price range.
Long-Term Trading Strategies
Long-term traders seek to profit from market trends that unfold over months or years. Some popular long-term strategies include:
- Buy and Hold: Buying assets and holding them for extended periods, focusing on long-term growth.
- Value Investing: Identifying undervalued assets and holding them until their market price corrects.
- Dollar-Cost Averaging (DCA): Investing a fixed amount regularly, regardless of market conditions, to reduce risk over time.
2. Algorithms for Different Trading Strategies
The next step is choosing the right trading algorithms for your strategy. Let’s explore algorithms for both day trading and long-term investing:
For Day Trading: Moving Average Crossover
The moving average crossover strategy is commonly used by day traders to capture trends by monitoring two moving averages—one short-term and one long-term:
import numpy as np
def moving_average_crossover(prices, short_window, long_window):
short_ma = prices.rolling(window=short_window).mean()
long_ma = prices.rolling(window=long_window).mean()
signals = np.where(short_ma > long_ma, 1, -1) # Buy when short MA crosses above long MA
return signals
For Long-Term Trading: Mean Reversion Strategy
The mean reversion strategy assumes that prices will revert to their mean over time, providing opportunities for long-term traders:
def mean_reversion_strategy(prices, lookback_window):
mean_price = prices.rolling(window=lookback_window).mean()
signals = np.where(prices < mean_price, 1, -1) # Buy if price is below mean, sell if above
return signals
3. Building an Automated Trading Bot with Interactive Brokers API
To automate trade execution, you can connect your trading bot to a broker like Interactive Brokers using their API. Let’s break down the steps:
Step 1: Set Up Interactive Brokers API
First, install the necessary Python libraries:
pip install ibapi
Step 2: Trading Example with Interactive Brokers API
Here's a basic example of setting up a connection and executing trades using Interactive Brokers’ API:
from ibapi.client import EClient
from ibapi.wrapper import EWrapper
from ibapi.contract import Contract
class TradingBot(EClient, EWrapper):
def __init__(self):
EClient.__init__(self, self)
def nextValidId(self, orderId):
self.start()
def start(self):
contract = Contract()
contract.symbol = 'AAPL'
contract.secType = 'STK'
contract.exchange = 'SMART'
contract.currency = 'USD'
self.reqMktData(1, contract, "", False, False, [])
def stop(self):
self.done = True
self.disconnect()
app = TradingBot()
app.connect("127.0.0.1", 7497, 0)
app.run()
4. Implementing AI (GPT-4) to Automate Trading
One of the most powerful tools you can add to your bot is GPT-4, which can analyze news, market sentiment, and even suggest trade decisions based on natural language processing.
Using GPT-4 for Sentiment Analysis
Here’s an example of how you can integrate GPT-4 to analyze sentiment for a specific stock:
import openai
def get_sentiment(stock_name):
prompt = f"Analyze the sentiment around {stock_name} in today's news headlines."
response = openai.Completion.create(
model="gpt-4",
prompt=prompt,
max_tokens=100
)
sentiment = response['choices'][0]['text']
return sentiment
Automating Trade Decisions Based on Sentiment
Once you have the sentiment analysis, you can make trading decisions accordingly:
sentiment = get_sentiment('AAPL')
if 'positive' in sentiment.lower():
print("Buy signal for AAPL")
else:
print("Hold/Sell signal for AAPL")
Conclusion: Automate Your Investments with AI
Building an AI investing bot can significantly enhance your trading strategy, whether you're day trading or focusing on long-term investments. By combining the power of traditional algorithms with advanced AI tools like GPT-4, you can automate decision-making and execute trades with precision. Start by defining your strategy, coding your bot, and connecting it to your brokerage account using the Interactive Brokers API. With AI automation, you'll be ahead of the curve in today's ever-evolving financial markets.